- Published on
Understanding Node Js Dependencies
- Authors
- Name
- Smail Oubaalla
- @smaiil_dev
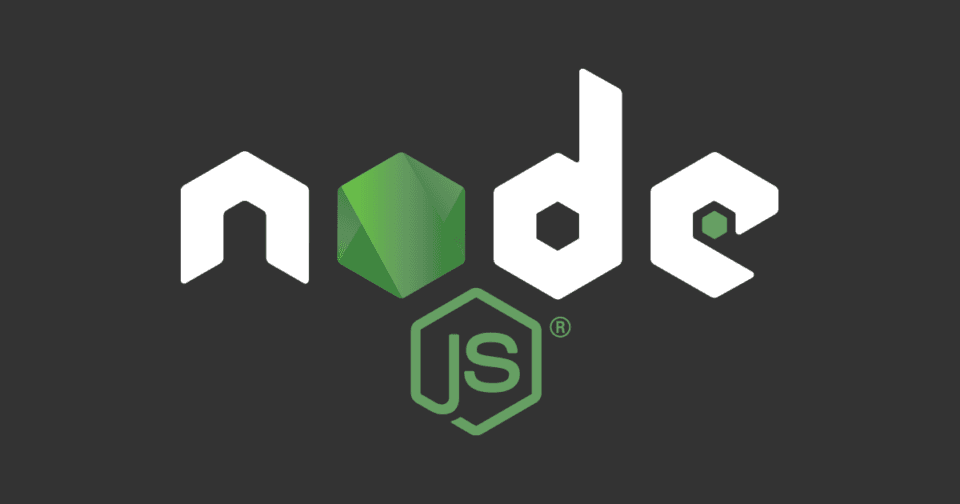
Why Dependencies Matter in Node.js
The Role of Dependencies
In the world of Node.js, dependencies are the building blocks of applications. Imagine constructing a building; rather than creating every brick from scratch, you use pre-made bricks that are proven to work. That's what dependencies do - they are pre-built modules that add specific functionalities to your application, saving you time and effort.
Why Not a Single Package?
Now, you might wonder, "Why not cram everything into one giant package?" Here’s the catch: Modularity is key. By using separate packages, you ensure that your application is lightweight, only including what it needs. This approach makes your app more efficient and easier to maintain. Plus, it's way easier to update or replace a single module than to untangle a spaghetti code mess in a monolithic package.
Understanding Node.js Dependencies
In Node.js, dependencies are managed through the package.json
file. This file categorizes dependencies into three main types:
- Dependencies: These are essential for your application to run.
- DevDependencies: These are used during development (like testing tools) but aren't needed in production.
- PeerDependencies: These are dependencies that are shared by multiple packages, ensuring compatibility and preventing conflicts.
NPM: Node.js' Package Manager
NPM (Node Package Manager) is the backbone of managing Node.js dependencies. It’s like a vast library where you can find and install different packages, and it takes care of version control and dependency resolution for you.
A Quick Look at Other Ecosystems
- .NET's NuGet: Similar to NPM, NuGet is the package manager for .NET, offering a plethora of libraries and tools for .NET developers.
- Python's PIP: Python uses PIP (Python Package Installer), which functions similarly, managing libraries and dependencies for Python applications.
In all these ecosystems, package managers play a pivotal role in simplifying dependency management, making developers' lives a bit easier.
What's Ahead?
Up next, we'll dive into the types of Node.js dependencies in more detail, and I'll share some insider tips on managing them like a pro. Stay tuned for a journey into the heart of Node.js efficiency!
Dependencies in Action: The "WeatherWiz" Project
Project Overview
"WeatherWiz" is our fictional Node.js application, designed to provide real-time weather information. It's a straightforward CLI (Command Line Interface) tool where users can enter a location and receive the current weather details.
Setting Up the Project
First, we initialize our project, creating a new directory and setting up a package.json
file:
mkdir WeatherWiz
cd WeatherWiz
npm init -y
Adding a Dependency
For fetching weather data, we add the axios package to our project. axios is a promise-based HTTP client, perfect for making API requests:
npm install axios
After this command, axios will be listed in our package.json under dependencies.
Using the Dependency
Here's how we use axios in our index.js file to fetch weather data:
const axios = require('axios');
async function getWeather(location) {
// Code to fetch and display weather data
}
getWeather('New York'); // Example usage
Understanding Dependency Bundling: The Case of Axios
Now that we've added axios
to our "WeatherWiz" project, it's crucial to understand how it fits into the bigger picture, especially when it comes to production.
Why Axios is Bundled for Production
Axios in Production:
axios
, as a dependency listed underdependencies
in ourpackage.json
, is not just for development; it's essential for the application's functionality in production. This is because our application relies onaxios
to fetch real-time weather data, a core feature of "WeatherWiz".Bundling Process: When we prepare our application for production, dependencies listed under
dependencies
are bundled along with our application. This ensures that all the necessary modules are included, and our app functions as intended in the production environment.Impact on Performance and Maintenance: Including
axios
as a bundled dependency means that our application will have everything it needs to run independently. This enhances the reliability and maintainability of the application, as we don't have to worry about external dependencies in the production environment.
The Significance of Correct Categorization
It's important to categorize your dependencies correctly in package.json
. Dependencies essential for the application to run in production, like axios
in our case, must be listed under dependencies
. This ensures they are included in the production build, maintaining the app's functionality and integrity.
Integrating a DevDependency: Jest in "WeatherWiz"
Adding Jest to Our Project
As our "WeatherWiz" project grows, we need to ensure its reliability. This is where testing comes in, and for that, we'll use jest
, a delightful JavaScript Testing Framework. Here's how we add Jest to our project as a development dependency:
npm install jest --save-dev
Running this command adds jest to our package.json, but under a different section - devDependencies
Why Jest is a DevDependency
Role in Development: jest is crucial for our development process, allowing us to write and run tests to ensure our code is bug-free and behaves as expected. However, it's not needed for the application to function in a production environment.
Not Bundled for Production: Unlike axios, jest is not bundled with our application when preparing for production. This is intentional, as jest is only used during the development phase for testing purposes.
Reducing Production Load: By not bundling jest in our production build, we keep our application lightweight and efficient. Production builds should contain only what is necessary for the application to run, ensuring optimal performance and reduced resource usage.
Correct Categorization in package.json
In our package.json, you'll now see jest listed under devDependencies. This categorization tells npm (and anyone reading our project's configuration) that jest is a dependency used exclusively for development. Here's how our package.json looks:
"devDependencies": {
"jest": "^VERSION_NUMBER"
}
This distinction between dependencies and devDependencies is a key aspect of effective Node.js project management.
With jest now part of our development toolkit, we've added another layer of professionalism and reliability to our "WeatherWiz" project. In the next section, we'll explore peerDependencies and their unique role in Node.js projects.
Understanding PeerDependencies: A Key Component in Node.js
Introducing PeerDependencies
As we continue to enhance our "WeatherWiz" project, it's time to explore a less frequently discussed but equally important aspect of Node.js dependencies: peerDependencies
.
What are PeerDependencies?
PeerDependencies
are a special kind of dependency in Node.js projects. They are used when a package needs to ensure compatibility with another package, but does not directly require it. This is common in plugins or extensions.
Why Use PeerDependencies?
- Ensuring Compatibility: The primary role of
peerDependencies
is to specify versions of a package that are compatible with your module. This prevents issues where incompatible versions of a package could potentially break your application. - Avoiding Redundancy:
peerDependencies
allow multiple packages to rely on a single shared dependency without each package having to include it separately. This reduces redundancy and keeps the overall project size smaller.
Example of a PeerDependency
Imagine our "WeatherWiz" project uses a plugin for axios
to add extra functionality. This plugin doesn't directly include axios
; instead, it lists axios
as a peerDependency
. This way, the plugin ensures that it's used with a compatible version of axios
already present in the project.
Here's how it might look in the package.json
:
"peerDependencies": {
"axios": "^0.21.1"
}
This entry indicates that the plugin is compatible with axios version 0.21.1 and above, but it relies on the project itself to have axios installed.
With this exploration of peerDependencies, our understanding of the Node.js dependency ecosystem deepens. We've now covered all three types of dependencies: dependencies, devDependencies, and peerDependencies, each playing a unique role in the development and functionality of Node.js applications.
In our next and final section, we'll wrap up everything we've learned about Node.js dependencies and conclude our deep dive into this essential aspect of Node.js project management
Conclusion: Mastering the Art of Node.js Dependencies
As we conclude our journey through the world of Node.js dependencies, let's reflect on the key insights we've gathered. Understanding the intricacies of dependencies
, devDependencies
, and peerDependencies
is not just a matter of good practice; it's an essential skill for any Node.js developer aiming for efficient, maintainable, and robust applications.
Key Takeaways
- Dependencies: The core building blocks of your application. They are included in your production build and are essential for your application's functionality.
- DevDependencies: Tools and utilities used during development, like testing frameworks or build tools. They streamline your development process but aren't needed in production.
- PeerDependencies: Ensure compatibility between shared modules, particularly important in modular and scalable applications.
The Importance of Proper Management
The way we manage these dependencies directly impacts the performance, size, and maintainability of our applications. Mismanagement can lead to bloated applications, version conflicts, or even production failures. But with the correct categorization and understanding of each dependency type, we set the stage for smooth development and deployment processes.
Embracing Continuous Learning
The Node.js ecosystem is dynamic, with new packages and updates being released constantly. Staying informed and adapting to these changes is part of the continuous learning journey in the tech world.
Final Thoughts
With our "WeatherWiz" project example, we've seen these concepts in action, providing a practical context to our discussion. Remember, the art of dependency management in Node.js is a blend of technical understanding and practical application, a skill honed over time and experience.
Thank you for joining me on this insightful exploration of Node.js dependencies. Here's to building more efficient, powerful, and well-managed Node.js applications!